Python Variables and Assignment Statements
Python variables are named memory locations (storage). They are like spreadsheet cells that store spreadsheet expressions (data values, formulas, and/or functions). However, a spreadsheet cell reveals the value produced by its expression. In a way, spreadsheet cells show both [program] code and output. Python variables are more explicit. One creates Python variables and sets data to the variables, using assignment statements that look like equations but that should not be confused with equations. Assignments consist of three parts: Left-hand Side (LHS), Equal Sign (ES), and Right-hand Side (RHS). The LHS is a Python variable and the RHS is an expression.
LHS | ES | RHS |
variable_name | = |
expression |
First, the
RHS expression is evaluated. If it is valid, its value is assigned to the
LHS variable. If there is a problem with the
RHS expression, an exception is raised and the program is terminated unless it is properly handled (see
exception handling in
Python). Thus a
Python assignment is a data transfer (from
right to
left).
Some other languages indicate data transfer (assignment) more explicitly. For example, R can implement the first statement as:
annual_rate <- 0.048
Like spreadsheet cells, Python variables are given their data types by example, based on the RHS expression.
Numeric Variables
Example 1 shows Python assignment statements, involving numeric variables.
Example 1 |
LHS | ES | RHS |
annual_rate | = |
0.048 |
monthly_rate | = | annual_rate/12 |
Spreadsheets consist of thousands of pre-defined variables that are referred to as cells. They are assigned generic "names", consisting of column and row references (locations). For example, cell C2 defines a variable at the intersection of column C and row 2. One can also give specific [meaningful] names the spreadsheet cells. Using the generic references, Example 1 can be implemented in Excel as follows:
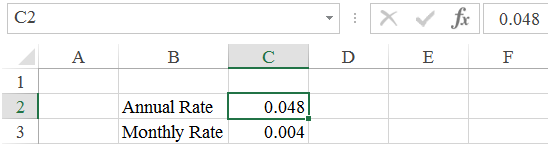 |
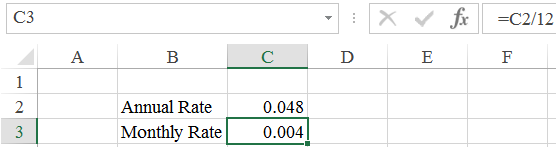 |
Figure 1 Using generic references for two spreadsheet assignments. |
Notice that labels "Annual Rate" and "Monthly Rate", stored in cells B2 and B3, serve a descriptive purpose (annotating cells next to them). The assignment statements, shown in Example 1, are implemented directly in cells C2 and C3. One can interpret them as: "C2 is set to 0.048" and "C3 divides the value of C2 by 12". In a more compact way, these assignments can be defined as:
C2: 0.048
C3: =C2/12
Using the same reference (variable) names in Python, the statements would look as:
C2 = 0.048
C3 = C2/12
With cells C2, C3 named as annual_rate and monthly_rate, respectively, this spreadsheet will take the following form:
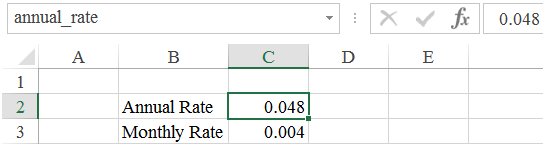 |
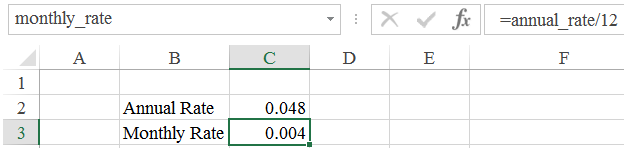 |
Figure 2 Using named references for two spreadsheet assignments. |
Again, a more compact spreadsheet notation would be:
annual_rate: 0.048
monthly_rate: =annual_rate/12
Using the same reference (variable) names in Python, the statements will look like in Example 1:
annual_rate = 0.048
monthly_rate = annual_rate/12
There is a very important difference between the spreadsheet statements and Python statements. The spreadsheet cell, C2, holding value 0.048 acts as a variable (named as annual_rate). Cell B2 holds label "annual rate:", which serves as an annotation, providing the meaning of the cell C2 content. In Python, a variable is created when placed on the left-hand side (LHS). It is reused on the right-hand side (RHS) in other expressions.
String Variables
Example 2 shows Python assignment statements, involving string variables.
Example 2 |
LHS | ES | RHS |
first_name | = | "Ann" |
last_name | = | "Annson" |
Python strings are arrays of bytes standing for UNICODE characters. Strings can be combined, using a "+" operator.
You can access any part of a string, using index array expressions. For example, to
full_name = first_name + " " + last_name
full_name
'Ann Annson'
To extract part of a string, use an array indexed expression. For example to get the first name from variable full_name, we do this:
fn = full_name[0:3]
fn
'Ann'
Notice that index range i:j makes index i inclusive and index j—exclusive. Thus expression full_name[0:3] gets a substring of string full_name from position 0 to position 2.
What about getting the last name?
ln = full_name[4:10]
ln
'Annson'
The above string extraction example is kind of primitive. We know the location of the space separating the first name from the last name. We would have to change the code if we wanted to process a different full name. Let find out the location of the space (k) and the string size (n) programmatically and, using this information, extract the first and last names.
k = full_name.find(" ")
n = len(full_name)
Notice that to find the location of the space, we use method find and to calculate the length of the string, we use function len. Now we can do more universal extractions (assuming that there is just one space, separating the first and last names).
fn = full_name[0:k]
fn
'Ann'
ln = full_name[k+1:n]
ln
'Annson'
To check this procedure for another string, try this:
astronomer = "Mikołaj Kopernik"
k = astronomer.find(" ")
n = len(astronomer)
fn = astronomer[0:k]
fn
'Mikołaj'
ln astronomer[k+1:n]
ln
'Kopernik'
Even more powerful solution can be worked out with method split that breaks down a string into substrings separated with one or more spaces.
names = astronomer.split()
fn = names[0]
fn
'Mikołaj'
ln = names[1]
ln
'Kopernik'